The THREADS package was converted to use Windows "fibres" and C++ a few years ago. However, fibres did not seem sufficiently general, so this package is now in process of being converted to use Boost, to take advantage of Boost multithreading. The earlier, fibres-based, version is still available as a zip file.
Contents
- General
- Component Coding
- Network Definitions
General
CppFBP is an enhanced version of the older C-based THREADS software, and has two different ways of defining networks:
- "fixed form" - this defines a network as a linked set of C structures. Unlike the JavaFBP and C#FBP conventions, these are not the control blocks used by CppFBP to run, but are used to initialize them at run-time. This separation is intended to allow changes to be made to the CppFBP internals, without requiring all users to change their network definitions.
- "free form" - this is a text-based notation, originally devised by Wayne Stevens, and now generally referred to as ".fbp notation", or the FBP DSL. The NoFlo project has adopted this notation, but also allows end-of-line to indicate the end of a "clause" (in addition to the comma).
A program is included, named Thxgen
, which can be used to convert free form network definitions into (runnable) fixed form definitions. However, the free form notation can also be run directly, if you are willing to pay the price of decoding the free form every time.
Sample Component
Under Visual C++ 2010 Express, for components, you will need to add the CppFBPCore/Headers file to Configuration Properties/C/C++/Additional Include Directories - plus $(LUA_INCLUDE) if you are going to be working with Lua.
For networks, you will need to add the CppFBPCore/lib file to Configuration Properties/C/C++/Additional Include Directories for your component - plus $(BOOST_INCLUDE). In addition, you will need to add the CppFBPCore/lib file and and CppFBPComponents/lib file to Configuration Properties/Linker/Additional Library Directories - plus $(BOOST_LIB).
Here is a simple component written in C++ to copy IPs from IN to
OUT.
Let's call this rather unoriginally ThCopy
.
|
The first action is always to initialize the port table using a call
to dfsdfpt
- the 2nd parameter of the call to dfsdfpt
must match the dimension declared for the port table. This number must
also match the number of port names specified in the parameters to dfsdfpt
. Note: dfsdfpt
modifies the port table, so this must not
be defined as constant.
The return statement causes deactivation (but not necessarily termination, as described above). The return code value on the return statement determines whether the process is willing to be reactivated: a value of 5 or greater forces termination even if data is available.
API Calls
Here are the API calls available to components in CppFBP:
Create a Packet:
|
All the port-related calls now have a character form, where the port can be referenced using a character string - this entails some extra overhead at run-time, but facilitates interfacing with scripting languages such as Lua. In these cases, the original service name is suffixed with a 'c'.
Parameters:
Note that the ptr, size, type
and port table element
parameters all have &
's attached, except in the case of dfscrep
, where only ptr
has it. The &
's are required
because
these parameters may be modified, and C parameters are all passed by
value
(except for strings and arrays). In some cases a particular service
does
not set them, but for consistency &
's are used for all of
them
(except dfscrep
).
port_count
and elem_no
are all binary integer
variables
(int
). size
is binary long. dfscrep
is
restricted
to a maximum of 64000 bytes.
-
proc_anchor
: a variable of type anchor (defined inthxanch.h
) -
ptr
: a void pointer used to point at IPs -
size
: a long variable containing the IP's size -
type
: a null-terminated string of up to 32 chars -
port_count
: parameter todfsdfpt
- must match the dimension of the definedport_tab
array for this component -
elem_no
: this specifies the element of the appropriate port to be used in a send, receive or close. These are only required for array-type ports, where they number up from 0. For non-array ports, this parameter must be 0. -
port_tab
: this parameter is declared as an array of typeport_ent
(see below), where each element corresponds to one of the ports known to the component; the whole structure is passed todfsdfpt
, while specific elements ofport_tab
are passed tosend
,receive
andclose
using&
. -
port_name_n
: port name to be used bydfsdfpt
; the number of these must match theport_count
parameter
After a call to dfsdfpt
, elem_count
in each port_ent
instance will
be set to the number of connected elements for that port, and ret_code
will
be set to 0 or 2, depending on whether that port was connected or not.
Service return codes:
dfscrep:
|
Limitations:
There is a limit of 4000 elements per array port.
Working storage for a component should not exceed a few thousand
bytes (including the working storage of any subroutines it calls). If
the component needs more than this, it should use one of C's dynamic
allocation functions (e.g. malloc
or calloc
) to
allocate the additional storage.
Network Notation
Fixed-Format Definition
As stated above, CppFBP supports two network definition notations: fixed form, and free form.
Here is an example of a network in fixed form notation. Note: the above structures are not the internal control blocks of CppFBP - they are an encoding of the free-form network specification notation. This separation will allow CppFBP to be extended in the future without requiring network definitions to be reprocessed.
#include "thxdef.h" #include
|
An exclamation mark ("!") in the upstream process name of a connection (cnxt_ent
) indicates an IIP-type connection.
An asterisk ("*") as the port name of a connection indicates an automatic port (Chapter 13 of the 2nd Edition).
Free-Format Definition
The free-form alternative notation, an early form of which is described briefly in Chapter 22 of the 2nd edition, basically follows a "flow" style, where connections can be chained together until a process has no output ports connected. This constitutes the end of a "clause", and is indicated by a comma or end-of-line (in both NoFlo and CppFBP).
This free form notation can be converted to the fixed form notation, using Thxgen
, or can be executed directly by CppFBP (interpretive mode).
Here is an example:
'data.fil'->OPT Reader(THFILERD) OUT -> IN Selector(THSPLIT) MATCH -> ... ,
|
The general syntax for free-form network definitions is quite simple, and can be shown as follows (using a variant of the notation which has started to become popular for defining syntax):
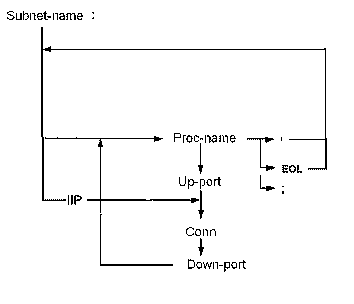
"EOL" ("end of line") indicates the alternative NoFlo convention for "end of clause". The mark above "EOL" is meant to be a comma.
Other symbols:
- "Proc-name" represents a process name, optionally followed by the component name (in round brackets)
- "Conn" represent an arrow, with optional capacity (in round brackets), e.g.
(30)
- "IIP" represents a quoted string (using single quotes) - any internal quotes must be escaped
- "Up-port" and "down-port" are from the point of view of the connection - they could also be called "output port" and "input port", respectively
An asterisk ("*") as the port name of a connection indicates an automatic port (Chapter 13 of the 2nd Edition).
The main network may be followed by one or more subnets, which have
basically the same notation (each one starting with a label and
finishing
with a semi-colon). However, subnets have to have additional notation
describing
how their external port names relate to their internal ones. Since this
association
is like an equivalence, we use the symbol =>
to indicate
this relationship. Thus,
, port-name-1 => port-name-2 process-A port-name-3,
|
indicates that port-name-1
is an external input port of
the subnet,
while port-name-2
is the corresponding input port of
process-A. Similarly,
, port-name-1 process-A port-name-2 => port-name-3,
|
indicates that port-name-3
is an external output port of
the subnet, while port-name-2
is the corresponding output
port of process-A.
The component name can be specified on any occurrence of the process name. This can then be followed optionally by a question mark to indicate that tracing is desired.
In Chapter 12 of the 2nd edition we alluded to the fact that we can specify that a
process "must run at least once" - this is specified by means of an
attribute file for the component, which has the name of the component,
and an extension
of atr
, e.g. thcount.atr
. These files must be
provided by the supplier of
a component, and must have a specific format. So far, only the "must
run"
attribute has been defined - it is specified by coding one of the
character
strings must_run
, Must_run
or MUST_RUN
,
with optional preceding blanks, in
the attribute file for a given component. If no attribute file is found
for a component, default values are assumed to apply (the default for
the
"must run" attribute is "need not run").
The fixed form network definition notation is a data-only C++ program, and specifies a network,
together
with all referenced subnets. Its importance is that this will be the
source
form for networks owned by customers, so CppFBP must guarantee that
any
enhancements to it will be upwards compatible. These guarantees are
essentially
encoded in the C headers which it uses: thxiip.h
, thxanch.h
and thxscan.h
.